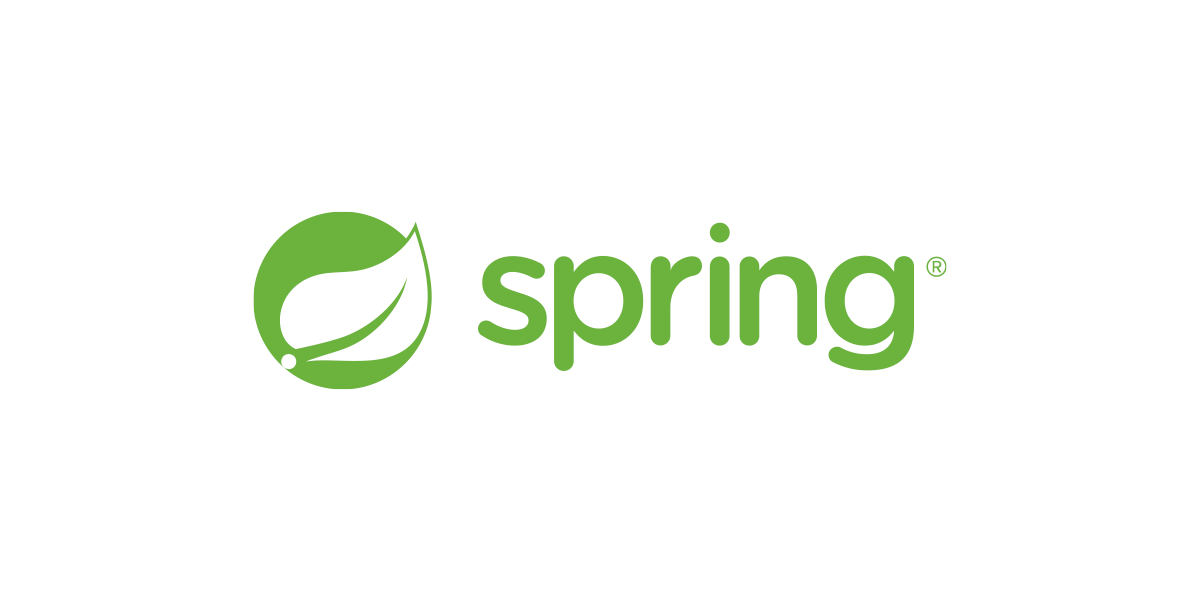
Table of Contents
[스프링인액션] JMX로 스프링 모니터링
스프링인액션 18장을 읽고 스프링 모니터링에 대해 정리하였다.
- 액추에이터 엔드포인트 MBeans 사용하기
- 스프링 빈을 MBeans로 노출
- 알림 발행(전송)하기
에 대해 작성하였다.
MBeans 사용하기
자바 어플리케이션을 모니터링하고 관리하는 표준 방법으로 JMX(Java Management Extensions)가 존재한다. JMX 클라이언트는 MBeans(managed beans) 라는 컴포넌트를 노출함으로써 오퍼레이션 호출, 속성 검사, MBeans의 이벤트 모니터링을 수행한다.
/heapdump를 제외한 모든 엑추에이터 엔드포인트는 MBeans로 노출되어 있다. 따라서 어떤 JMX 클라이언트를 사용해도 액추에이터 엔드포인트 MBeans와 연결할 수 있다. MBeans는 HTTP 처럼 명시적으로 포함시키지 않아도 기본적으로 노출된다.
노출되는 엔드포인트 설정
application.yml 설정 파일에 노출하거나 제외할 엔드포인트를 설정하면 된다.
1management:
2 endpoints:
3 jmx:
4 exposure:
5 include: health, info, bean, conditions
6 exclude: env, metrics
노출할 때는 include 설정을, 노출에서 제외할 때는 exclude 를 설정한다.
MBenas 생성
빈 클래스에 @ManagedResource 어노테이션을 지정하고, 메서드에는 @ManagedOperation, 속성에는 @ManagedAttribute만 지정해주면 어떤 빈도 JMX MBeans로 쉽게 노출할 수 있다.
1@Service
2@ManagedResource
3public class TacoCounter extends AbstractRepositoryEventListener<Taco> {
4
5 private AtomicLong counter;
6
7 public TacoCounter(TacoRepository tacoRepo) {
8 long initialCount = tacoRepo.count();
9 this.counter = new AtomicLong(initialCount);
10 }
11
12 @Override
13 protected void onAfterCreate(Taco entity) {
14 counter.incrementAndGet();
15 }
16
17 @ManagedAttribute
18 public long getTacoCount() {
19 return counter.get();
20 }
21
22 @ManagedOperation
23 public long increment(long delta) {
24 return counter.addAndGet(delta);
25 }
26}
클래스 인스턴스에 @ManagedResource 를 지정하여 이 빈이 MBeans도 된다는 것을 나타낸다. @ManagedAttribute로 지정한 getTacoCount() 메서드는 MBeans 속성으로 노출되고, @ManagedOperation 으로 지정한 increment() 메서드는 MBeans 오퍼레이션으로 노출된다.
알림 전송
기본적으로 MBeans 오퍼레이션과 속성은 pull 방식을 사용하므로 MBeans 값이 변경되더라도 자동으로 알려주지 않는다. 그러나 스프링의 NotificationPublisher를 사용하여 MBeans는 JMX 클라이언트에 알림을 push 할 수 있다.
1@Service
2@ManagedResource
3public class TacoCounter extends AbstractRepositoryEventListener<Taco>
4 implements NotificationPublisherAware {
5
6 private AtomicLong counter;
7 private NotificationPublisher np;
8
9 @Override
10 public void setNotificationPublisher(NotificationPublisher np) {
11 this.np = np;
12 }
13
14 @ManagedOperation
15 public long increment(long delta) {
16 long before = counter.get();
17 long after = counter.addAndGet(delta);
18 if ((after / 100) > (before / 100)) {
19 Notification notification = new Notification("taco.count", this, before, after + "th taco created!");
20 np.sendNotification(notification);
21 }
22 return after;
23 }
24}
MBeans가 알림을 발행하려면 NotificationPublisherAware 인터페이스의 setNotificationPublisher() 메서드를 구현하면 된다. 위의 예시는 NotificationPublisherAware 인터페이스를 구현하고, 주입된 NotificationPublisher를 사용하여 100개의 객체가 생성될 때마다 알림을 전송하는 코드이다.
Posts in this Series
- [스프링인액션] JMX로 스프링 모니터링
- [스프링인액션] 스프링 관리
- [스프링인액션] 스프링 액추에이터 사용
- [스프링인액션] 실패와 지연 처리
- [스프링인액션] 클라우드 구성 관리
- [스프링인액션] 마이크로서비스 이해
- [스프링인액션] 리액티브 데이터 퍼시스턴스
- [스프링인액션] 리액티브 API 개발
- [스프링인액션] 리액터 개요
- [스프링인액션] 스프링 통합 플로우 사용
- [스프링인액션] 비동기 메시지 전송하기 - Kafka
- [스프링인액션] 비동기 메시지 전송하기 - RabbitMQ
- [스프링인액션] 비동기 메시지 전송하기 - JMS
- [스프링인액션] REST API 사용하기
- [스프링인액션] REST API 생성하기
- [스프링인액션] 구성 속성 사용
- [스프링인액션] 스프링 시큐리티
- [스프링인액션] 데이터로 작업하기
- [스프링인액션] 스프링 초기 설정